Build an E-Commerce assistant with Query Agents
Sign up here for notifications on Weaviate Agents, or visit this page to see the latest updates and provide feedback.
In this tutorial, we will be building a simple e-commerce assistant agent with the Weaviate Query Agent. This agent will have access to multiple Weaviate collections, and will be capable of answering complex queries about brands and clothing items, accessing information from each collection.
We've prepared a few public datasets that you can use to explore the Query Agent. They are available on HuggingFace:
- E-Commerce: A dataset that lists clothing items, prices, brands, reviews, etc.
- Brands: A dataset that lists clothing brands and information about them, such as their parent brand, child brands, average customer rating, etc.
Introduction: What are Query Agents?
The Weaviate Query Agent is a pre-built agentic service designed to answer natural language queries based on the data stored in Weaviate Cloud. The user simply provides a prompt/question in natural language, and the Query Agent takes care of all intervening steps to provide an answer.
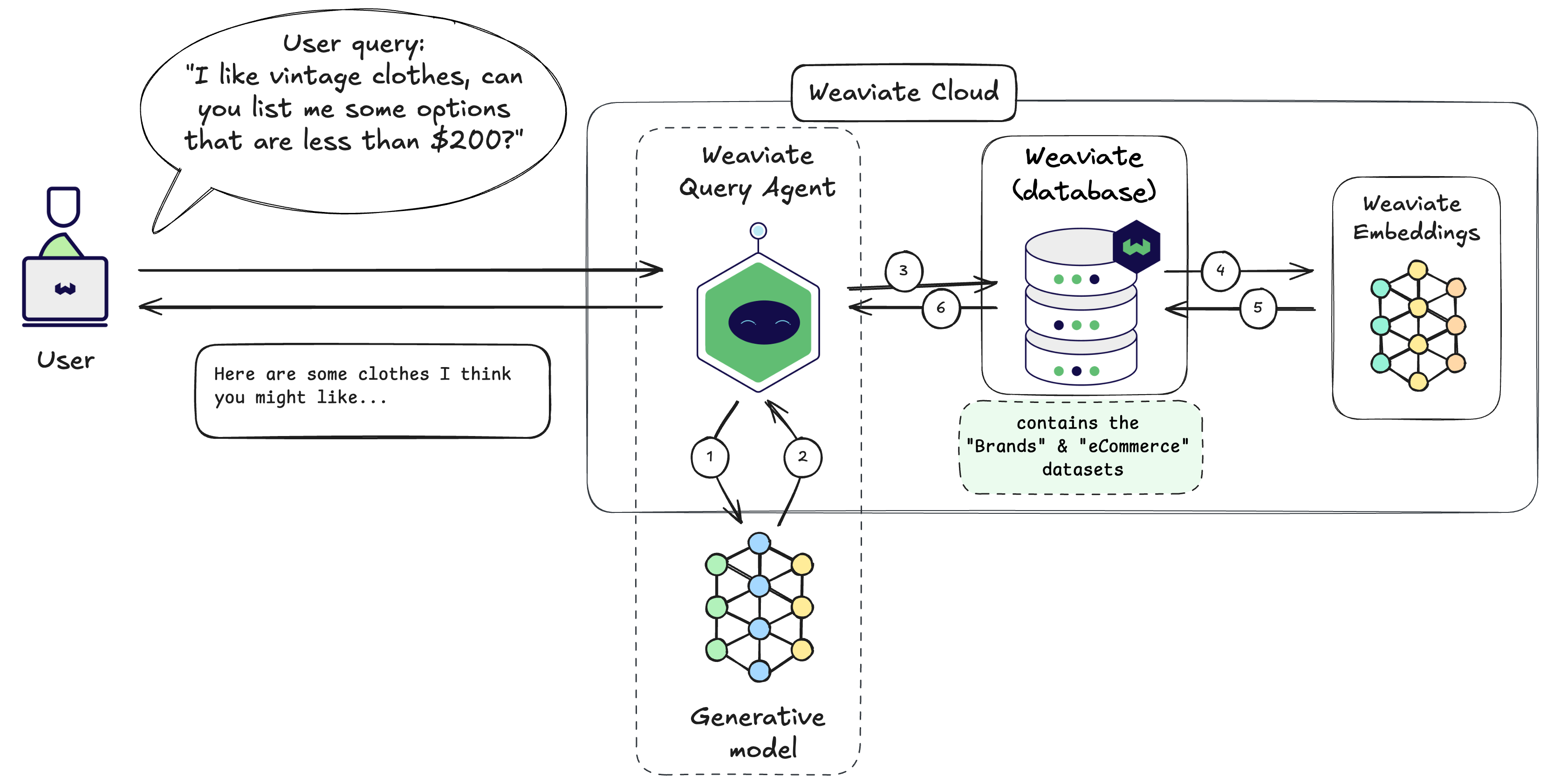
The Query Agent:
- Receives a task in the form of a question or instructions.
- Uses an appropriate generative model (e.g. large language model) to analyze the task and determine the exact queries to perform.
- Sends the queries to Weaviate, which vectorizes the queries as needed using the specified vectorizer integration (we will use Weaviate Embeddings).
- Receives the results from Weaviate and uses appropriate generative models to generate the final response to the user prompt/query.
Prerequisites
To use the Weaviate Agents and Weaviate Embedding service, you need to have a Weaviate Cloud account.
Step 1: Set up Weaviate
Now, let's get started by setting up a Weaviate Cloud instance that we will use for this tutorial and connecting it to the Python client.
1.1 Create a Weaviate Cloud cluster
- Create a free Sandbox cluster in Weaviate Cloud.
- Take note of the
REST Endpoint
andAdmin
API key to connect to your cluster. (for more info, check out the quickstart)
In this tutorial, we are using the Weaviate Embeddings service as the vectorizer, so you do not have to provide any extra keys for external embedding providers. Weaviate Embeddings uses the Snowflake/snowflake-arctic-embed-l-v2.0
as the default embedding model.
If you want to use another vectorizer, check out the list of supported model providers.
1.2 Install the Python libraries
In order to install the Weaviate Python client together with the agents
component, run:
- Python
pip install "weaviate-client[agents]"
You will also need datasets
, a lightweight library providing access to the publicly hosted datasets on HuggingFace.
- Python
pip install datasets
Troubleshooting: Force pip
to install the latest version
For existing installations, even pip install -U "weaviate-client[agents]"
may not upgrade weaviate-agents
to the latest version. If this occurs, additionally try to explicitly upgrade the weaviate-agents
package:
- Python
1.3 Connect to your instance
Now, you can finally connect to your Weaviate Cloud instance with the parameters from the first step:
- Python Client v4
import os
import weaviate
from weaviate.auth import Auth
# Best practice: store your credentials in environment variables
weaviate_url = os.environ["WEAVIATE_URL"]
weaviate_api_key = os.environ["WEAVIATE_API_KEY"]
client = weaviate.connect_to_weaviate_cloud(
cluster_url=weaviate_url,
auth_credentials=Auth.api_key(weaviate_api_key),
)
print(client.is_ready()) # Should print: `True`
# Your work goes here!
client.close() # Free up resources
After running this snippet, you should see the message True
printed out, which means that you have successfully connected to your instance.
Step 2: Prepare the Collections
In the following code blocks, we are pulling our demo datasets from Hugging Face and writing them to new collections in our Weaviate Sandbox cluster. Before we can start importing the data into Weaviate, we need to define the collections, which means setting up the data schema and choosing the vectorizer/embedding service.
2.1 Define the Collections
Below you can see what the objects in the datasets E-Commerce
and Brands
look like.
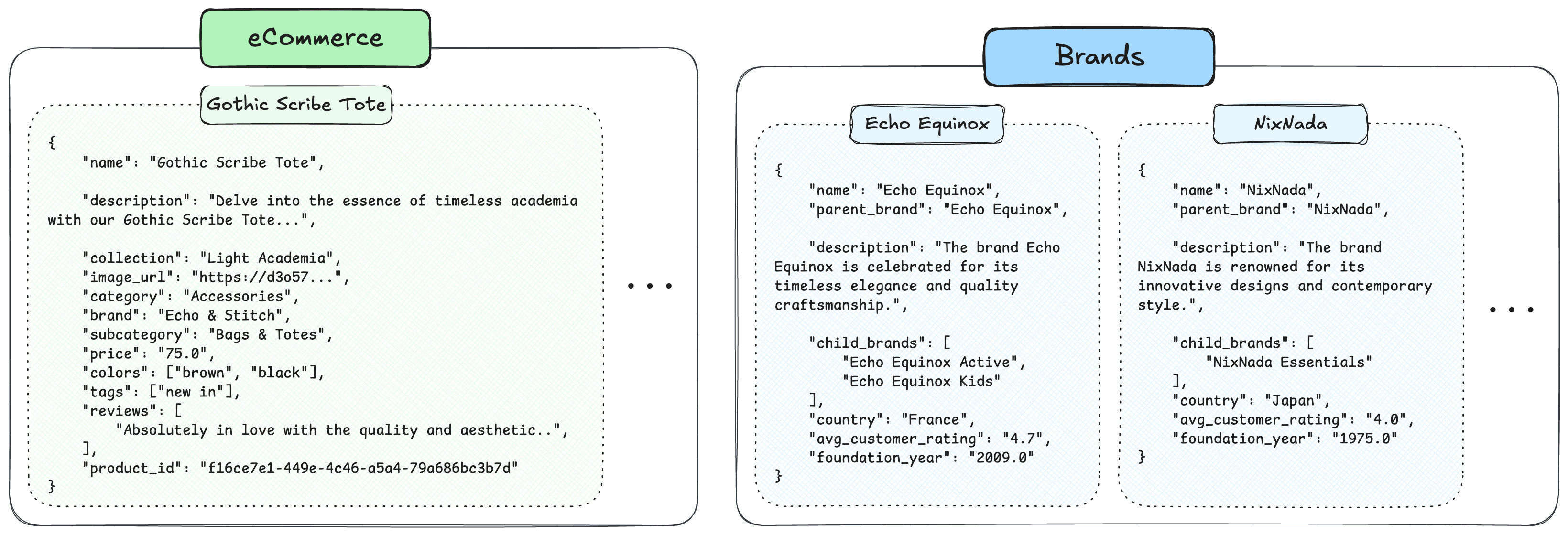
For the collection Brands
, we are going to use the auto-schema
option which creates properties automatically based on the imported data.
However, this is not the best way of importing the data.
The Query Agent uses the descriptions of collections and properties to decide which ones to use when solving queries. You can experiment by changing these descriptions and providing more details. This is why it's recommended to manually define the data schema and add the property descriptions.
For example, below we make sure that the Query Agent knows that prices are all in USD, which is information that would otherwise be unavailable.
- Python Client v4
from weaviate.classes.config import Configure, Property, DataType
# Using `auto-schema` to infer the data schema during import
client.collections.create(
"Brands",
description="A dataset that lists information about clothing brands, their parent companies, average rating and more.",
vectorizer_config=Configure.Vectorizer.text2vec_weaviate(),
)
# Explicitly defining the data schema
client.collections.create(
"ECommerce",
description="A dataset that lists clothing items, their brands, prices, and more.",
vectorizer_config=Configure.Vectorizer.text2vec_weaviate(),
properties=[
Property(name="collection", data_type=DataType.TEXT),
Property(
name="category",
data_type=DataType.TEXT,
description="The category to which the clothing item belongs",
),
Property(
name="tags",
data_type=DataType.TEXT_ARRAY,
description="The tags that are assocciated with the clothing item",
),
Property(name="subcategory", data_type=DataType.TEXT),
Property(name="name", data_type=DataType.TEXT),
Property(
name="description",
data_type=DataType.TEXT,
description="A detailed description of the clothing item",
),
Property(
name="brand",
data_type=DataType.TEXT,
description="The brand of the clothing item",
),
Property(name="product_id", data_type=DataType.UUID),
Property(
name="colors",
data_type=DataType.TEXT_ARRAY,
description="The colors on the clothing item",
),
Property(name="reviews", data_type=DataType.TEXT_ARRAY),
Property(name="image_url", data_type=DataType.TEXT),
Property(
name="price",
data_type=DataType.NUMBER,
description="The price of the clothing item in USD",
),
],
)
2.2 Populate the database
Now, we can import the pre-vectorized data (E-Commerce and Brands) into our Weaviate Cloud instance:
- Python Client v4
from datasets import load_dataset
brands_dataset = load_dataset(
"weaviate/agents", "query-agent-brands", split="train", streaming=True
)
ecommerce_dataset = load_dataset(
"weaviate/agents", "query-agent-ecommerce", split="train", streaming=True
)
brands_collection = client.collections.get("Brands")
ecommerce_collection = client.collections.get("ECommerce")
with brands_collection.batch.fixed_size(batch_size=200) as batch:
for item in brands_dataset:
batch.add_object(properties=item["properties"], vector=item["vector"])
with ecommerce_collection.batch.fixed_size(batch_size=200) as batch:
for item in ecommerce_dataset:
batch.add_object(properties=item["properties"], vector=item["vector"])
failed_objects = brands_collection.batch.failed_objects
if failed_objects:
print(f"Number of failed imports: {len(failed_objects)}")
print(f"First failed object: {failed_objects[0]}")
print(f"Size of the ECommerce dataset: {len(ecommerce_collection)}")
print(f"Size of the Brands dataset: {len(brands_collection)}")
By calling len()
on our collections, we can check that the import has successfully concluded and see what the size of our collections is.
Size of the E-Commerce dataset: 448
Size of the Brands dataset: 104
Step 3: Set up the Query Agent
3.1 Basic Query Agent
When setting up the query agent, we have to provide a few things:
- The
client
- The
collection
which we want the agent to have access to. - (Optionally) A
system_prompt
that describes how our agent should behave - (Optionally) Timeout - which for now defaults to 60s.
Let's start with a simple agent. Here, we're creating an agent
that has access to our Brands
and ECommerce
datasets.
- Python Client v4
from weaviate.agents.query import QueryAgent
agent = QueryAgent(
client=client,
collections=["ECommerce", "Brands"],
)
And that's about it! Now, you can start using the Query Agent.
3.2 Customized Query Agent (with system prompt)
In some cases, you may want to define a custom system_prompt
for your agent. This can help you provide the agent with some default instructions for how to behave. For example, let's create an agent that will always answer the query in the user's language.
- Python Client v4
multi_lingual_agent = QueryAgent(
client=client,
collections=["ECommerce", "Brands"],
system_prompt="You are a helpful assistant that always generates the final response in the user's language."
"You may have to translate the user query to perform searches. But you must always respond to the user in their own language.",
)
We will use this custom agent multi_lingual_agent
in step 4.5.
Step 4: Run the Query Agent
When we run the agent, it will first make a few decisions depending on the query:
- The agent will decide which collection (or multiple collections) to look up an answer in.
- The agent will also decide whether to perform a regular search query, what filters to use, whether to do an aggregation query, or all of them together.
- It will then provide a response within
QueryAgentResponse
.
4.1 Ask a question
Let's start with a simple question:
- "I like vintage clothes, can you list me some options that are less than $200?"
- Python Client v4
response = agent.run(
"I like vintage clothes, can you list me some options that are less than $200?"
)
response.display()
The response from the agent is:
original_query='I like vintage clothes, can you list me some options that are less than $200?' collection_names=['ECommerce'] searches=[[QueryResultWithCollection(queries=['vintage clothes'], filters=[[IntegerPropertyFilter(property_name='price', operator=<ComparisonOperator.LESS_THAN: '<'>, value=200.0)]], filter_operators='AND', collection='ECommerce')]] aggregations=[] usage=Usage(requests=3, request_tokens=7681, response_tokens=428, total_tokens=8109, details=None) total_time=11.588264226913452 aggregation_answer=None has_aggregation_answer=False has_search_answer=True is_partial_answer=False missing_information=[] final_answer='Here are some vintage-inspired clothing options under $200:\n\n1. **Vintage Philosopher Midi Dress ($125.00)** - A deep green velvet fabric dress with antique gold button detailing, perfect for an academic or sophisticated setting. [Echo & Stitch]\n\n2. **Vintage Gale Pleated Dress ($120.00)** - A burgundy pleated dress capturing the Dark Academia aesthetic, great for poetry readings or contemplative afternoons. [Solemn Chic]\n\n3. **Retro Groove Flared Pants ($59.00)** - Electric blue flared pants capturing early 2000s millennial pop culture chic. [Vivid Verse]\n\n4. **Vintage Scholar Tote ($90.00)** - A deep green canvas tote with brown leather accents, ideal for carrying scholarly essentials. [Echo & Stitch]\n\n5. **Retro Futura Tee ($29.98)** - A bold graphic tee with early 2000s pop culture references, perfect for a nostalgic throwback. [Vivid Verse]\n\n6. **Electric Velvet Trousers ($60.00)** - Neon green velvet trousers with a flared leg, inspired by turn-of-the-century fashion icons. [Vivid Verse]\n\n7. **Vintage Ivy Loafers ($120.00)** - Burgundy leather loafers offering timeless sophistication, suitable for academic settings. [Solemn Chic]' sources=[Source(object_id='6040c373-9cce-421d-8f4e-e01346cbf29f', collection='ECommerce'), Source(object_id='06a83da4-92ff-4cbc-9cc6-c55fc6ed49b9', collection='ECommerce'), Source(object_id='a5a0927d-2859-47e6-8455-d77e0190aa53', collection='ECommerce'), Source(object_id='a5f3c394-d207-4064-a2bc-b9f655d09ddc', collection='ECommerce'), Source(object_id='5d7bb719-2df5-4a7c-9bbc-275450ab29c6', collection='ECommerce'), Source(object_id='50f4c89c-faf9-4430-b393-c39ede5d511d', collection='ECommerce'), Source(object_id='5b6d5a1f-e218-4400-ac9f-df59466f3c97', collection='ECommerce')]
But this output is a bit too much, so let's just return the field final_answer
:
- Python Client v4
# Only print the final answer of the response
print(response.final_answer)
The output is:
Here are some vintage-inspired clothing options under $200:
1. **Vintage Philosopher Midi Dress ($125.00)** - A deep green velvet fabric dress with antique gold button detailing, perfect for an academic or sophisticated setting. [Echo & Stitch]
2. **Vintage Gale Pleated Dress ($120.00)** - A burgundy pleated dress capturing the Dark Academia aesthetic, great for poetry readings or contemplative afternoons. [Solemn Chic]
3. **Retro Groove Flared Pants ($59.00)** - Electric blue flared pants capturing early 2000s millennial pop culture chic. [Vivid Verse]
4. **Vintage Scholar Tote ($90.00)** - A deep green canvas tote with brown leather accents, ideal for carrying scholarly essentials. [Echo & Stitch]
5. **Retro Futura Tee ($29.98)** - A bold graphic tee with early 2000s pop culture references, perfect for a nostalgic throwback. [Vivid Verse]
6. **Electric Velvet Trousers ($60.00)** - Neon green velvet trousers with a flared leg, inspired by turn-of-the-century fashion icons. [Vivid Verse]
7. **Vintage Ivy Loafers ($120.00)** - Burgundy leather loafers offering timeless sophistication, suitable for academic settings. [Solemn Chic]
By using the display()
method on a QueryAgentResponse
, you can get a user-friendly output of the following information:
- 🔍 Original Query -
original_query
- 📝 Final Answer -
final_answer
- 🔭 Searches Executed -
searches
- 📊 Aggregations Run -
aggregations
- 📚 Sources -
sources
- ⚠️ Missing Information -
missing_information
- 📊 Usage Statistics -
usage
- Total Time Taken -
total_time
- Python Client v4
# Print the whole response in a user-friendly format
response.display()
The response is:
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔍 Original Query ────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ I like vintage clothes, can you list me some options that are less than $200? │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📝 Final Answer ─────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ Here are some vintage-inspired clothing options under $200: │
│ │
│ 1. **Vintage Philosopher Midi Dress ($125.00)** - A deep green velvet fabric dress with antique gold button detailing, perfect for an academic or sophisticated setting. [Echo & Stitch] │
│ │
│ 2. **Vintage Gale Pleated Dress ($120.00)** - A burgundy pleated dress capturing the Dark Academia aesthetic, great for poetry readings or contemplative afternoons. [Solemn Chic] │
│ │
│ 3. **Retro Groove Flared Pants ($59.00)** - Electric blue flared pants capturing early 2000s millennial pop culture chic. [Vivid Verse] │
│ │
│ 4. **Vintage Scholar Tote ($90.00)** - A deep green canvas tote with brown leather accents, ideal for carrying scholarly essentials. [Echo & Stitch] │
│ │
│ 5. **Retro Futura Tee ($29.98)** - A bold graphic tee with early 2000s pop culture references, perfect for a nostalgic throwback. [Vivid Verse] │
│ │
│ 6. **Electric Velvet Trousers ($60.00)** - Neon green velvet trousers with a flared leg, inspired by turn-of-the-century fashion icons. [Vivid Verse] │
│ │
│ 7. **Vintage Ivy Loafers ($120.00)** - Burgundy leather loafers offering timeless sophistication, suitable for academic settings. [Solemn Chic] │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔭 Searches Executed 1/1 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ QueryResultWithCollection(queries=['vintage clothes'], filters=[[IntegerPropertyFilter(property_name='price', operator=<ComparisonOperator.LESS_THAN: '<'>, value=200.0)]], filter_operators='AND', collection='ECommerce') │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ 📊 No Aggregations Run │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭─────────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📚 Sources ────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ - object_id='6040c373-9cce-421d-8f4e-e01346cbf29f' collection='ECommerce' │
│ - object_id='06a83da4-92ff-4cbc-9cc6-c55fc6ed49b9' collection='ECommerce' │
│ - object_id='a5a0927d-2859-47e6-8455-d77e0190aa53' collection='ECommerce' │
│ - object_id='a5f3c394-d207-4064-a2bc-b9f655d09ddc' collection='ECommerce' │
│ - object_id='5d7bb719-2df5-4a7c-9bbc-275450ab29c6' collection='ECommerce' │
│ - object_id='50f4c89c-faf9-4430-b393-c39ede5d511d' collection='ECommerce' │
│ - object_id='5b6d5a1f-e218-4400-ac9f-df59466f3c97' collection='ECommerce' │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
📊 Usage Statistics
┌────────────────┬──────┐
│ LLM Requests: │ 3 │
│ Input Tokens: │ 7681 │
│ Output Tokens: │ 428 │
│ Total Tokens: │ 8109 │
└────────────────┴──────┘
Total Time Taken: 11.59s
4.2 Ask a follow-up question
The agent can also be provided with additional context. For example, we can provide the previous response as context and get a new_response
- Python Client v4
new_response = agent.run(
"What about some nice shoes, same budget as before?", context=response
)
new_response.display()
The response is:
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔍 Original Query ────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ What about some nice shoes, same budget as before? │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📝 Final Answer ─────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ Here are some great vintage-style shoes priced under $200 from various collections that blend style with nostalgia: │
│ │
│ 1. **Vintage Noir Loafers** ($125): These dress shoes, part of the Dark Academia collection, come in black and grey, offering a timeless look with subtle modern twists. They have received positive reviews for style and comfort, once broken │
│ in (Source: 1). │
│ │
│ 2. **Vintage Ivy Loafers** ($120): Another classic from the Dark Academia line, these deep burgundy loafers combine elegance with comfort, as appreciated by users in their reviews (Source: 2). │
│ │
│ 3. **Glide Platforms** ($90): For a more playful option, these high-shine pink platform sneakers offer a nod to the Y2K era, receiving praise for their comfort and unique look (Source: 3). │
│ │
│ 4. **Celestial Step Platform Sneakers** ($90): With a dreamy sky blue color, these Y2K-inspired sneakers provide comfort and a touch of whimsy (Source: 4). │
│ │
│ 5. **Garden Stroll Loafers** ($90): From the Cottagecore collection, these cream loafers feature delicate floral motifs for a chic, countryside-inspired look (Source: 5). │
│ │
│ 6. **Bramble Brogues** ($120): In dusky green, these suede brogues add pastoral elegance to any outfit, admired for their craftsmanship (Source: 7). │
│ │
│ 7. **Garden Fête Heels** ($125): These cottagecore heels feature floral embroidery for a touch of elegance perfect for garden parties (Source: 10). │
│ │
│ 8. **Garden Gala T-Straps** ($125): Elegant cream and sage T-strap heels featuring floral embellishments, perfect for a whimsical yet classy touch (Source: 18). │
│ │
│ All these options fall under the $200 budget, offering a variety of styles from classic and elegant to colorful and playful, accommodating different fashion tastes and preferences. │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔭 Searches Executed 1/1 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ QueryResultWithCollection(queries=['vintage shoes'], filters=[[IntegerPropertyFilter(property_name='price', operator=<ComparisonOperator.LESS_THAN: '<'>, value=200.0)]], filter_operators='AND', collection='Ecommerce') │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────── 📊 Aggregations Run 1/1 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ AggregationResultWithCollection( │
│ search_query=None, │
│ groupby_property=None, │
│ aggregations=[IntegerPropertyAggregation(property_name='price', metrics=<NumericMetrics.MEAN: 'MEAN'>)], │
│ filters=[TextPropertyFilter(property_name='subcategory', operator=<ComparisonOperator.LIKE: 'LIKE'>, value='vintage')], │
│ collection='Ecommerce' │
│ ) │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭─────────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📚 Sources ────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ - object_id='92efe1bb-77ee-4002-8618-4c9d7c3972c2' collection='Ecommerce' │
│ - object_id='f51001bf-c0c6-47d5-b165-4e7ce878900e' collection='Ecommerce' │
│ - object_id='9ba9ed81-c917-4e13-8058-d6ad81896ef3' collection='Ecommerce' │
│ - object_id='41f324c2-a8e8-4cde-87d0-42661435a32c' collection='Ecommerce' │
│ - object_id='d8d8e59e-d935-4a6f-9df1-bc2477452b11' collection='Ecommerce' │
│ - object_id='505238e0-9993-4838-89fc-513c8f53d9f8' collection='Ecommerce' │
│ - object_id='4a1321dc-d3f5-405d-8b21-e656c448ccfd' collection='Ecommerce' │
│ - object_id='b59f7700-2633-43d6-807e-72dda35d545c' collection='Ecommerce' │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
📊 Usage Statistics
┌────────────────┬───────┐
│ LLM Requests: │ 5 │
│ Input Tokens: │ 15998 │
│ Output Tokens: │ 906 │
│ Total Tokens: │ 16904 │
└────────────────┴───────┘
Total Time Taken: 22.77s
4.3 Perform an aggregation
Now, let's try a question that requires an aggregation. Let's see which brand lists the most shoes.
- Python Client v4
response = agent.run("What is the the name of the brand that lists the most shoes?")
response.display()
The response is:
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔍 Original Query ────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ What is the the name of the brand that lists the most shoes? │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📝 Final Answer ─────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ The brand that lists the most shoes is Loom & Aura, with a total count of 118 shoes across various styles such as Cottagecore, Fairycore, Goblincore, Light Academia, and Dark Academia. │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ 🔭 No Searches Run │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────── 📊 Aggregations Run 1/1 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ AggregationResultWithCollection( │
│ search_query=None, │
│ groupby_property='brand', │
│ aggregations=[TextPropertyAggregation(property_name='collection', metrics=<TextMetrics.TOP_OCCURRENCES: 'TOP_OCCURRENCES'>, top_occurrences_limit=1)], │
│ filters=[], │
│ collection='ECommerce' │
│ ) │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
📊 Usage Statistics
┌────────────────┬──────┐
│ LLM Requests: │ 3 │
│ Input Tokens: │ 5794 │
│ Output Tokens: │ 238 │
│ Total Tokens: │ 6032 │
└────────────────┴──────┘
Total Time Taken: 7.52s
4.4 Search over multiple collections
In some cases, we need to combine the results of searches across multiple collections. From the result above, we can see that "Loom & Aura" lists the most shoes.
Let's imagine a scenario where the user would now want to find out more about this company, as well as the items that they sell.
- Python Client v4
response = agent.run(
"Does the brand 'Loom & Aura' have a parent brand or child brands and what countries do they operate from? "
"Also, what's the average price of a shoe from 'Loom & Aura'?"
)
response.display()
The response is:
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔍 Original Query ────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ Does the brand 'Loom & Aura' have a parent brand or child brands and what countries do they operate from? Also, what's the average price of a shoe from 'Loom & Aura'? │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📝 Final Answer ─────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ The brand "Loom & Aura" operates as a parent brand with several child brands, including "Loom & Aura Active," "Loom & Aura Kids," "Nova Nest," "Vivid Verse," "Loom Luxe," "Saffrom Sage," "Stellar Stitch," "Nova Nectar," "Canvas Core," and │
│ "Loom Lure." "Loom & Aura" itself is based in Italy and operates in multiple countries where its child brands are located, such as the USA, Japan, South Korea, Spain, the UK, and France. │
│ │
│ The average price of a shoe from "Loom & Aura" is approximately $87.11, based on the aggregation of available data on shoe prices. │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔭 Searches Executed 1/2 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ QueryResultWithCollection(queries=['Loom parent brand and child brands', 'Aura parent brand and child brands'], filters=[[], []], filter_operators='AND', collection='Brands') │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭──────────────────────────────────────────────────────────────────────────────────────────────────────────── 🔭 Searches Executed 2/2 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ QueryResultWithCollection(queries=['countries where Loom & Aura operates'], filters=[[TextPropertyFilter(property_name='name', operator=<ComparisonOperator.EQUALS: '='>, value='Loom & Aura')]], filter_operators='AND', collection='Brands') │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭───────────────────────────────────────────────────────────────────────────────────────────────────────────── 📊 Aggregations Run 1/1 ─────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ AggregationResultWithCollection( │
│ search_query='', │
│ groupby_property=None, │
│ aggregations=[IntegerPropertyAggregation(property_name='price', metrics=<NumericMetrics.MEAN: 'MEAN'>)], │
│ filters=[TextPropertyFilter(property_name='brand', operator=<ComparisonOperator.EQUALS: '='>, value='Loom')], │
│ collection='Ecommerce' │
│ ) │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
╭─────────────────────────────────────────────────────────────────────────────────────────────────────────────────── 📚 Sources ────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╮
│ │
│ - object_id='cb022de3-36ac-4ab9-94c6-cddbbebbb035' collection='Brands' │
│ │
╰───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────╯
📊 Usage Statistics
┌────────────────┬───────┐
│ LLM Requests: │ 5 │
│ Input Tokens: │ 11158 │
│ Output Tokens: │ 608 │
│ Total Tokens: │ 11766 │
└────────────────┴───────┘
Total Time Taken: 16.12s
4.5 Use a system prompt
We are going to use the custom agent from step 3.2 with the system prompt that says:
"You are a helpful assistant that always generates the final response in the user's language."
"You may have to translate the user query to perform searches. But you must always respond to the user in their own language."
For example, try to ask if the brand "Eko & Stitch" has a branch or associated company in the UK but using Japanese instead of English:
- Python Client v4
response = multi_lingual_agent.run('"Eko & Stitch"は英国に支店または関連会社がありますか?')
print(response.final_answer)
The response is:
Eko & Stitchは、英国に拠点があります。1974年に設立され、品質の高いクラフトマンシップとタイムレスなエレガンスで知られています。関連ブランドとして「Eko & Stitch Active」と「Eko & Stitch Kids」があります。また、Eko & Stitchの親会社はNova Nestです。
Or translated to English:
Eko & Stitch is based in the UK. It was established in 1974 and is known for its high-quality craftsmanship and timeless elegance. It has associated brands, "Eko & Stitch Active" and "Eko & Stitch Kids." Additionally, the parent company of Eko & Stitch is Nova Nest.
Summary
This guide shows you how to build an end-to-end e-commerce assistant using Weaviate's agentic services — from setting up your Weaviate Cloud instance and importing datasets to configuring a Query Agent that intelligently handles natural language queries.
The Query Agent automatically interprets queries to decide whether to run standard searches, filters, or aggregations. It connects to multiple collections and selects the right source based on your query. The agent can also use previous interactions to provide more relevant follow-up answers and you can define system prompts to ensure the agent always responds in the user’s preferred language or style.
Further resources
Questions and feedback
The official changelog for Weaviate Agents can be found here. If you have feedback, such as feature requests, bug reports or questions, please submit them here, where you will be able to see the status of your feedback and vote on others' feedback.
If you have any questions or feedback, let us know in the user forum.